
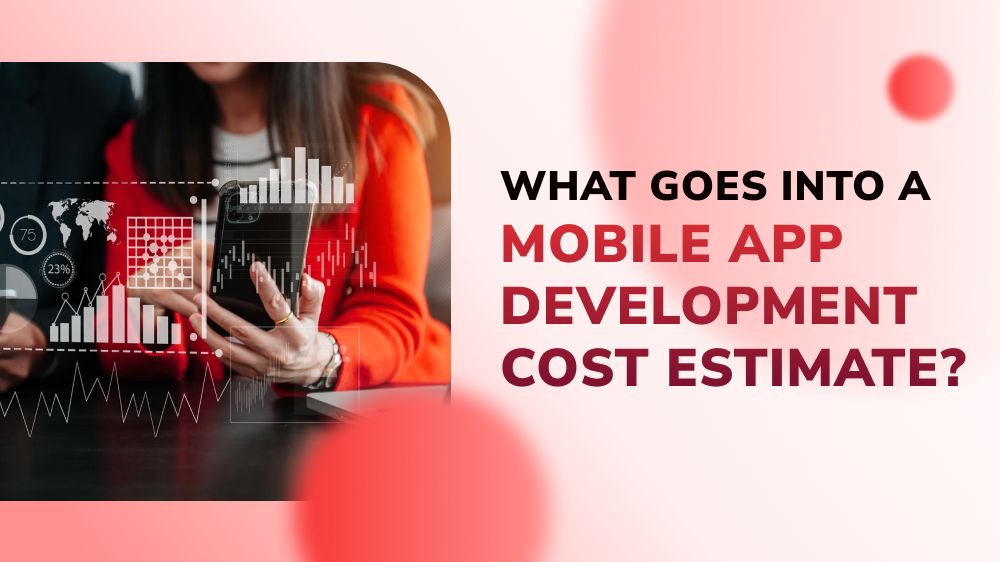
What Goes into a Mobile App Development Cost Estimate?
Planning on outsourcing your mobile app project? Find out what goes into a mobile app development cost estimate and how to choose the right developer.
Have you ever heard of reactive programming? It is a design approach that uses asynchronous programming to manage real-time updates to content.
Reactive programming is a new and challenging concept. There is also a lot of noise surrounding it, making it difficult for a beginner. In today’s article, we attempt to clear up any confusion surrounding this concept, especially when it comes to Java reactive programming. We will take a comprehensive dive into its definition, benefits, libraries, use cases, and potential challenges.
Before we dig deeper into other topics like reactive libraries or use cases, we would like to go over the important concepts of reactive programming. It is essential that you fully grasp them before moving on with the article.
Reactive programming, unlike the traditional programming paradigm, works with streams of data by reacting to events instead of waiting for them to happen. It is a type of programming that works with asynchronous data streams in a non-blocking way. In other words, reactive programming is the use of asynchronous data streams that send data to the consumer as it is available so that developers can design code that reacts in an asynchronous manner.
Java reactive programming is a programming approach that emphasizes the development of applications that are both responsive and scalable, capable of handling both concurrent and asynchronous operations effectively.
Reactive programming is a step towards a reactive system. Since reactive programming and reactive systems are such a large and challenging concept, a group of developers has come up with a manifesto for it. In 2013, the Reactive Manifesto was created in order to spell out the core principles of reactive programming. There are four fundamental characteristics that a reactive system must have: Responsive, resilient, elastic, and message-driven.
After understanding what reactive programming is, the essential follow-up question is why. Why is it beneficial to understand such a complex concept? The short answer to this question is that responsive applications have become a necessity. They are no longer a luxury. The longer answer to this question involves the multiple benefits of reactive programming:
Java is a popular language. Not only does it have a great collection of open-source libraries and a large supportive community, but Java is also utilized to build a variety of applications.
Seeing how reactive programming is essential for building resilient and scalable applications, it is beneficial to dig a little deeper and understand how Java reactive programming works. In other words, how does a programmer implement reactive programming in Java?
In Java, reactive programming is achieved through the use of Reactive Streams. Reactive Stream is a specification that defines a collection of interfaces and classes for building reactive applications. Thanks to this standard, several libraries and frameworks have been developed in Java to implement the Reactive Streams specification, including RxJava, Project Reactor, and Akka. These libraries provide developers with APIs for writing reactive code and creating reactive applications that are both scalable and responsive.
RxJava is a popular Reactive library for Android developers. It’s known as the “go-to” framework for making it easier to handle concurrency and asynchronous tasks that come with mobile programming. RxJava is an open-source Functional Reactive Programming (FRP) library for Android. It adds a layer of abstraction over threading to ease the implementation of complex concurrent behavior.
The Reactive library, Project Reactor, is a non-blocking application library for the Java Virtual Machine (JVM) that is derived from the Reactive Streams Specification. The Project Reactor library serves as the base for the reactive stack within the Spring ecosystem, and its development is in close cooperation with Spring.
Akka is a framework and runtime for building high-concurrency, distributed, fault-tolerant applications. Akka supports reactive programming by using the Actor model for concurrency,
The Akka framework supports the development of reactive applications using a wide range of programming models, from functional to declarative and imperative programming. The Akka framework supports Java and Scala for building reactive applications.
Spring Framework 5 uses Reactive streams as a way to communicate backpressure between asynchronous components and libraries in the framework. This is a specification that has been developed through industry collaboration and is also used in Java 9. The Spring Framework already supports Reactor internally, but now it’s using Reactor to extend it even more. For developers who are already familiar with Spring, it builds on Reactor and offers a similar programming model.
Reactive programming in Java is a powerful way to build high-performance, distributed, and responsive applications that meet the needs of today’s distributed and dynamic computing environments. It is a top choice when it comes to high-load or multi-user applications. The following are some of its popular use cases.
Although Java reactive programming seems like a must-have for building modern applications, there are still several challenges and disadvantages. Other than the fact that it shouldn’t be applied when there isn’t a heavy load or a large number of concurrent users modifying data, developers should also be aware of the following challenges:
All in all, Java reactive programming is a great tool for building robust, scalable, and responsive modern applications. It is natural for developers to experiment with new programming paradigms and strive for further development. Even then, try to keep in mind the difficulties that come along with learning and mastering new technological skills and carefully consider if it is the right solution for your projects.
You can always seek professional help and consultations to make the best decisions for your projects. Orient Software is one such experienced and credible team that is happy to help - whether you wish to apply Java reactive programming to your web application development mobile application development or if you are looking for an offshore development center that has mastered reactive coding in Java. Contact us and let us know what you need! Outlines checked. You can start writing the articles. Thanks.
Planning on outsourcing your mobile app project? Find out what goes into a mobile app development cost estimate and how to choose the right developer.
Is your business missing out on a secret weapon? Explore the REAL benefits of mobile apps for business and unlock explosive growth!
Take a look at the different types of healthcare software. Learn how these technologies are revolutionizing healthcare services.
Take a look at the best reading apps that are changing the way we consume content. Explore features and benefits, and find the perfect app for your reading needs.
Unsure of the best database for your iOS app? Compare SQLite, Core Data, and Realm to find the ideal match for your app’s needs.