21 Essential Software Engineering Practices
Step into the world of modern software engineering with this in-depth exploration of best practices. It reveals how development teams achieve excellence in every line of code.
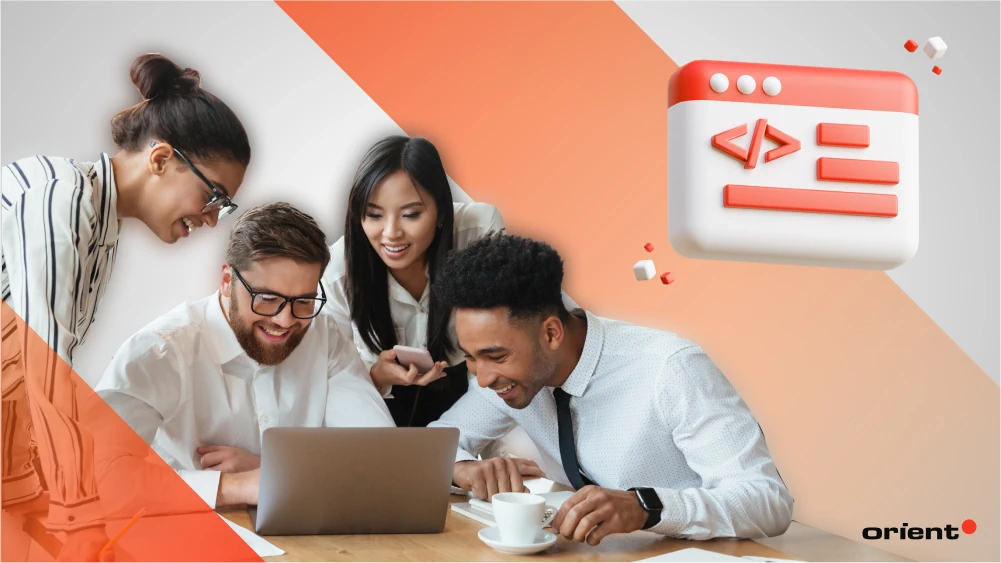
Content Map
More chaptersTypes of Software Engineering Practices
Here is a list of software engineering practices that will help your company deliver quality software.
Software engineers can learn best practices from different areas of software development. Learning best practices and then finding the right ways to apply them to a project matures software engineers and helps them succeed.
When choosing the set of best practices to focus on, a programmer’s experience matters. So while junior engineers usually learn best practices for writing unit tests or avoiding code smells, seniors or technical leaders should learn best practices that impact the entire project or development process.
It is essential to follow software engineering conventions to keep the software maintainable. Your organization will need to invest in these software engineering practices by training, coaching, tools, infrastructure, and time.
1. Development Methodologies To Consider
We have several development methodologies/frameworks to choose from, like Waterfall, Agile, Scrum, Nexus, and Kanban. However, to achieve the benefits of agile, you will need to change the way you think and execute your software projects.
Waterfall
Waterfall, for example, forces you to have plans and a big pile of requirements that you try to implement in a highly structured project, which takes much longer and consumes a lot more effort than it should.
Scrum
Scrum is a framework, a set of practices, that improve your quality, speed, and financial viability for software delivery projects. It’s not just the software development projects that benefit from it. Even product design and life cycle management can benefit from Scrum.
Nexus
Nexus helps scrum teams scale. Scaling agile to large and long-running projects that will deliver incremental product releases over time is challenging. Since it is often a lack of experience in teams to do developing at scale, it is better to concentrate on known and proven practices, such as Scrum. Nexus takes the ideas of Scrum and puts them into a framework to scale with up to more than a hundred developers.
Kanban
Kanban works by visualizing the amount of work required to deliver a new feature and then prioritizing and committing tasks to completion. In this process, developers oversee the project status reports. The value of such a practice is that it gives developers visibility of their status. You should choose the methodology that fits your organization.
2. Coding Practices: Code Readability & Clean Code
How readable the code is, is often considered one of the highest quality features in software. Your organization is responsible for ensuring your team reads each other’s code and commits only quality code. Poor code readability leads to problems such as bugs and less stable software, and you may have to rewrite part of your software, which will affect your team’s velocity. Make sure your team learns about clean coding practices.
3. Apply Refactoring Frequently
Software refactoring is the process of modifying or restructuring existing code to make it easier to understand, easier to maintain, and easier to change. For example, you might change the name of a method or how it is called. Refactoring allows you to add flexibility to your system to meet changing requirements or accommodate future code changes. Refactoring involves understanding existing code and requirements, restructuring the code for simpler maintenance, making changes to existing code to achieve the required flexibility, validating the change against requirements, and finally putting the change into production.
4. Reduce Your Technical Debt
Technical debt is used in the software industry to cover debt due to bugs, legacy code, or missing documentation. It is typically used interchangeably with design debt or code debt. When development teams take actions to expedite the delivery of a piece of functionality or project, they take on technical debt, which later needs to be refactored. Technical debt is the result of prioritizing speedy delivery over perfect code. To build a better product is to consider your existing code as debt. Refactoring and debt reduction will enable your organization to put your current customers first and make sure your debt is as small as possible. In addition, your code must be easy to understand, maintainable, bug-free, and reliable.
5. Coding Practices: KISS, YAGNI, DRY, and SOLID
These are some of the many acronyms in software.
KISS stands for “Keep It Simple, Stupid.” Often, developers make things more complex than they need to be. You should prefer simple solutions over advanced solutions if the simple solution fulfills the requirements.
YAGNI, “You Aren’t Gonna Need It;” developers often make things too general to cater to future requirements, and this is usually not a good idea.
DRY, “Don’t Repeat Yourself,” reminds us that duplicate code leads to more code, and more code is harder and more expensive to maintain.
Finally, the SOLID acronym stands for Single responsibility principle, Open-closed principle, Liskov substitution principle, Interface segregation principle, and Dependency inversion principle. If you follow these coding principles, you will produce better code that is easier to maintain.
6. Unit Test Your Code
Unit tests are the number one practice when building software. Unit testing will expose potential issues early in the development process. In most projects, relying only on manual testing would be impossible due to the complexity of software and the frequent release cycles. You need to unit test most of the software you write. When the automatic unit test fails, you know right away what is wrong.
7. Behavior Driven Development
Software development is often a time-consuming and expensive process, and communication between engineers and business professionals can be a bottleneck to project progress. Engineers often misunderstand what the business needs from its software, and business professionals often misunderstand the capabilities of their technical team.
BDD, or Behaviour-Driven Development, is a form of development that focuses on the behavior and expectations of the software’s users. It can be divided into two parts: writing examples in ubiquitous language to illustrate user behaviors and using those examples as automated tests. That ensures that functionality and the business’s vision for the system are maintained throughout development.
8. Automated Acceptance Testing
An acceptance test is a formal specification of how a software product should act and is expressed as a usage scenario or use case.
Automated acceptance testing is an integral part of a continuous delivery strategy. Developers must own the responsibility to make sure that these automated tests are passing and running smoothly.
By describing the acceptance test with BDD, you will be able to run automated acceptance test suites in DevOps software such as CircleCI, Jenkins, or Azure DevOps.
9. Performance Testing
Performance testing is the study and testing of the performance of an application as it meets certain criteria such as load or response time under specific conditions. Performance testing is often the last step before providing the software to an end-user. Don’t skip this step, as your users will not be happy with slow or unresponsive software.
10. Test-Driven Development
Test-Driven Development (TDD) is a development practice that helps you improve the software quality and time to market by continuously and automatically running through various user-defined tests to understand the functional behavior of the software that your team is creating. This process is one of the practices of software engineering that help handle the software product development process and improving the team’s overall working process.
11. Continuous Integration / Continuous Deployment
The agile software development approach requires every team member to integrate and deploy software changes to production. And this happens at least two times a day and every day. In addition, you need to make sure that every team member can track and fix problems quickly. These practices cover the whole software development life cycle, from coding/code reviewing to deployment/testing.
12. Software Architecture - Microservices
There is a growing trend of building software as a set of microservices. In general, a microservice is a simple building block that can be combined with other building blocks to form a complex system.
The primary advantage of using microservices is their flexibility, which is a direct consequence of separating functionality into small pieces. So, if you do not wish to maintain a monolith, adopting microservices is an option for you.
13. Software Architecture - Monoliths
A monolithic application structure is a good approach for applications that do not need microservices’ modular architecture. With monoliths, it is still essential to focus on separation of concerns (SoC). The main idea is to separate the functional concerns into different modules. Doing microservices right can be tricky, so sometimes, it makes sense to start with a monolith and refactor it into microservices if needed.
14. DevOps
DevOps is a set of development and operations practices that have emerged over the last several years in the software development field to help teams deliver projects in a highly agile way by merging software development and operations. DevOps aims to deliver software applications faster by introducing automation in almost everything.
DevOps is a mindset. This mindset encourages a flexible approach towards the product development process, considering the requirements, risks, and constraints of the various stakeholders involved, customer requirements, and working to meet the expectations.
15. Monitoring and Logging
Agile teams focus on continuous delivery, and doing so is challenging without monitoring and logging. You need to ensure that you can identify bugs in a controlled environment and trigger prompt corrective action for the development team. Observability is critical, so invest in a central monitoring and logging system like Elastic Stack, AWS CloudWatch, or Azure Monitor.
16. Infrastructure as Code
Infrastructure as code provides the ability to create, manage, and modify infrastructure in the cloud by using code. Terraform is one of the leading technologies for managing infrastructure as code. Infrastructure as code is important because you can then automate the infrastructure setup, which leads to more consistent and well-functioning infrastructure. You should store the infrastructure code in the version control system.
17. Configuration Management
Configuration management is the practice of configuring servers, applications, and infrastructure as code. There are many configuration management tools on the market, such as Ansible, Puppet, and Chef. You can use these technologies to configure servers and orchestrate automation tasks like installing the software for a database server, installing security patches to servers, and upgrading operating systems. Since these configurations are stored in code, it is testable and repeatable.
18. Cloud Computing
Developers will need to become familiar with platforms like Amazon Web Service, Microsoft’s Azure, and Google Cloud, as well as other cloud solutions like Apache’s CloudStack and OpenStack and IBM Cloud Orchestrator. Employees need training so they can maximize cloud development.
19. DevSecOps
It’s a software engineering practice dedicated to secure creation, delivery, and continuous software monitoring. So how can you create the most secure software by understanding security concerns and prioritizing risk-based cybersecurity strategies, and adapt fast to the fast-changing requirements of today’s software?
20. Pen-Testing
Pen-testing is testing for the vulnerabilities in software and hardware that your company will eventually use to deliver the service to your customers. The goal of pen-testing is to understand how your systems will respond to real-life problems before they arise and ensure that your systems are reliable and safe while implementing new features and functionality.
21. Communication and Collaboration
Communication and collaboration are crucial to achieving an agile approach. Having cross-functional teams, you need to ensure effective communication between team members and between individuals and the organization. Communication is done through writing and different mediums like face-to-face meetings, voice, video, social media, or conference calls. Communication will help team members work together, understand problems, solve problems, and know what to do next. People will start in a consensus-based culture and progress to peer-to-peer working, eventually moving into self-organizing groups to address identified business needs.
Conclusion
Agile software development practices have matured, and agile software delivery has become the way to go. As you iteratively develop your products, your ability to deliver higher quality at higher speeds will enable you to accomplish your company’s goals quicker.
But remember, it is not just the process that delivers your results, but the people who implement and apply the practices. Therefore, your business, technical, and management teams need to be fully engaged and committed to these practices to see the positive results from agile development practices.
If you don’t already have these software engineering practices in place, it’s time to start!